Python Strings Basics
Introduction to Python Strings
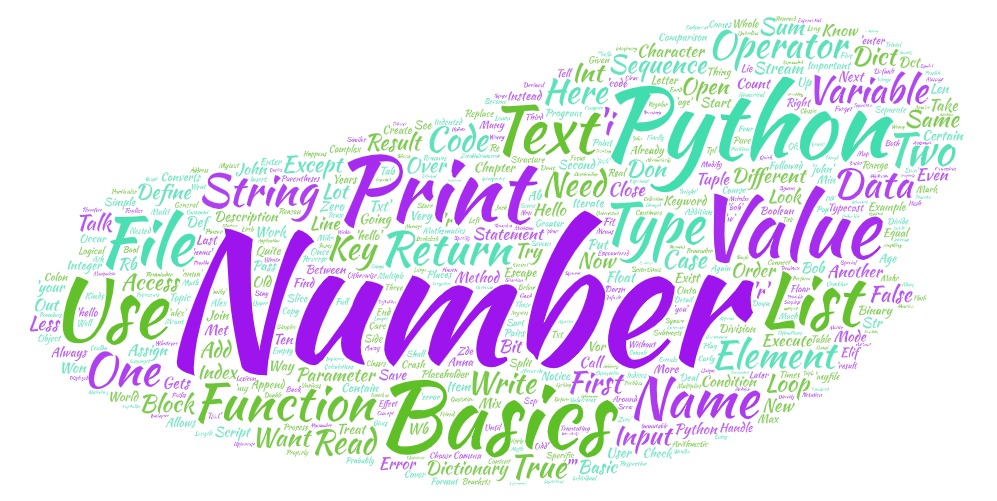
STRINGS
A string defines characters sequences. Strings always need to be surrounded by quotation marks. Otherwise the interpreter will not realize that they are meant to be treated like text. The keyword for String in Python is str .
Traversing a String
# Traversing
name = "Welcome"
for ch in name:
print(ch, '-', end = ' ')
# Reversing a string
## W - e - l - c - o - m - e -
name = "Reverse me"
# The Slice notation in python has the syntax -
# list[<start>:<stop>:<step>]
# So, when you do a[::-1], it starts from the end towards the first taking each element. So it reverses a. This is applicable for lists/tuples as well.
print(name[::-1])
## em esreveR
lgth = len(name)
for a in range(-1, (-lgth-1), -1):
print(name[a])
## Split the string into a list of characters, reverse the list, then rejoin into a single string
## e
## m
##
## e
## s
## r
## e
## v
## e
## R
print(''.join(reversed("Hello world")))
## dlrow olleH
Checking identities of two strings
You use == when comparing values and is when comparing identities.
lang = ['Java','Python']
more_lang = lang
print(lang == more_lang) # -> True
## True
print(lang is more_lang) # -> True
## True
even_more_lang = ['Java','Python']
print(lang == even_more_lang) #-> True
## True
print(lang is even_more_lang) #-> False
## False
print(id(lang))
## 842617096
print(id(more_lang))
## 842617096
print(id(even_more_lang))
## 865271048
Checking capital letters
The istitle() method checks if each word is capitalized.
print( 'The Hilman'.istitle() ) #=> True
## True
print( 'The Cat'.istitle() ) #=> False
## True
print( 'the rice'.istitle() ) #=> False
## False
Checking if string contains another
print( 'A' in 'The string containing A' ) #=> True
## True
print( 'Apple in' in 'The string containing A' ) #=> False
## False
Finding the index of a substring in a string
print('The'.find('The string containing A'))
## -1