React- JS
React JS Programming
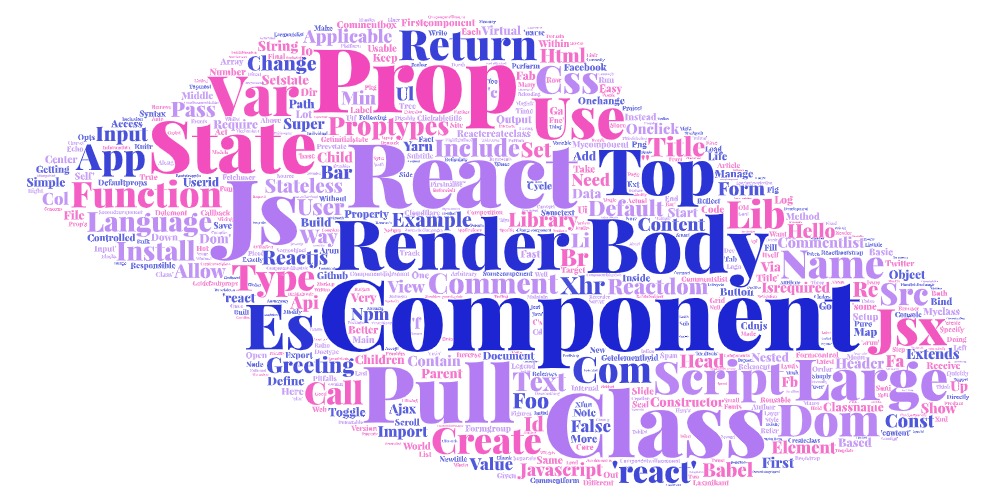
*** React-JS
Setup
first, we need to create our React application using create-react-app. Once that is done, you can proceed to create your first React component.
Install create-react-app globally by typing this command in your Terminal:
npm install -g create-react-app
Or you can use a shortcut:
npm i -g create-react-app
Build the App
Let’s build our first React application by following these steps:
Create our React application with the following command:
create-react-app my-first-react-app
Go to the new application with cd my-first-react-app and start it with npm start. The application should now be running at http://localhost:3000.
Create a new file called Home.js inside your src folder:
import React, { Component } from 'react';
class Home extends Component {
render() {
return <h1>I'm Home Component</h1>;
}
}
export default Home;
You may have noticed that we are exporting our class component at the end of the file, but it’s fine to export it directly on the class declaration, like this:
import React, { Component } from 'react';
export default class Home extends Component {
render() {
return <h1>I'm Home Component</h1>;
}
}
Now that we have created the first component, we need to render it. So we need to open the App.js file, import the Home component, and then add it to the render method of the App component. If we are opening this file for the first time, we will probably see a code like this in File: src/App.js
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
class App extends Component {
render() {
return (
<div className='App'>
<header className='App-header'>
<img src={logo} className='App-logo' alt='logo' />
<h1 className='App-title'>Welcome to React</h1>
</header>
<p className='App-intro'>
To get started, edit <code>src/App.js</code>
and save to reload.
</p>
</div>
);
}
}
export default App;
Let’s change this code a little bit. As I said before, we need to import our Home component and then add it to the JSX. We also need to replace the
element with our component, like this in File: src/App.js
import React, { Component } from 'react';
import logo from './logo.svg';
// We import our Home component here...
import Home from './Home';
import './App.css';
class App extends Component {
render() {
return (
<div className='App'>
<header className='App-header'>
<img src={logo} className='App-logo' alt='logo' />
<h1 className='App-title'>Welcome to React</h1>
</header>
{/* Here we add our Home component to be render it */}
<Home />
</div>
);
}
}
export default App;
As you can see, we imported React and Component from the React library. You probably noticed that we are not using the React object directly. To write code in JSX, you need to import React. JSX is similar to HTML, but with a few differences. In the following recipes, you will learn more about JSX.
This component is called a class component (React.Component), and there are different types: pure components (React.PureComponent) and functional components, also known as stateless components, which we will cover in the following recipes.
If you run the application, you should see something like this: